컴포넌트
prefetch
module.exports = {
chainWebpack: config => {
config.plugins.delete('prefetch'); //prefetch 삭제
}
}
import(/* webpackPrefetch: true*/ );
컴포넌
뷰 안에다가 만들 때 View.vue
컴포넌트 구조 이해하기
1. 컴포넌트
view data code의 세트라고 생각하고 실행
컴포넌트 안에 HTML 소스가 있고 이걸 실행하기 위해 자바스크립트 코드 데이터가 존재.
재사용이 가능해
2. snippet
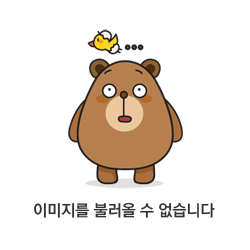
<template>
<div></div>
</template>
<script>
export default {
components: {}, // 다른 컴포넌트 사용 시 컴포넌트를 import하고, 배열로 저장
data() {
// HTML과 JS 코드에서 사용할 데이터 변수 선언
return {
sampleData: '',
};
},
setup() {}, // Composition API
created() {}, // 컴포넌트가 생성되면 실행
mounted() {}, // template에 정의된 HTML 코드가 렌더링된 후 실행
unmounted() {}, // unmount가 완료된 후 실행
methods: {}, // 컴포넌트 내에서 사용할 메소드 정의
};
</script>
vue.json
바디 안에
Generate Basic Vue Code 작성
"Generate Basic Vue Code" : {
"prefix": "vue-start",
"body" : [
"<template></template>\n<script>\nexport default {\n\tname:'',\n\tcomponents: {},\n\tdata() {\n\t\treturn {\n\t\t\tsampleData: ''\n\t\t};\n\t},\n\tbeforeCreate() {},\n\tcreated() {},\n\tbeforeMount() {}, \n\tmounted() {}, \n\tbeforeUpdate() {},\n\tupdated() {},\n\tbeforeMount() {},\n\tunmounted() {},\n\tmethods: {}\n}<script>"
],
"description": "Generate Basic Vue Code"
}
About vue에 붙여넣기
vue-start
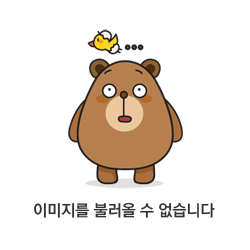
npm run serve를 하려고 보니 오류가 남
template 사이에 div 넣어줘야 하고
name: 대문자 소문자 섞어야
3. Lifecycle Hooks
모든 컴포넌트 생성될 때 초기화 단계 거쳐
화면 로딩 시간 개선 가능 사용자가 느끼는 체감 속도를 높임
A lifecycle hook provides a specified amount of time (one hour by default) to wait for the action to complete before the instance transitions to the next state.
데이터 바인딩
vue 양방향 데이터 바인딩 지원
모델에서 데이터를 정의한 후 뷰와 연결하면 어느 한 쪽에 변경이 일어났을 때 다른 한쪽에 자동으로 반영
①
<template>
<h1>Hello, {{title}}!</h1> <!-- * 주의 {{}} 중괄호 두 개!! -->
</template>
<script>
export default {
data() {
return{
title: 'World'
};
}
}
</script>
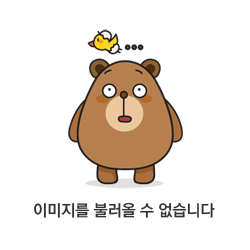
화면 보기 위해서
router.index.js에 import 해야 해
import { createRouter, createWebHistory } from 'vue-router'
import HomeView from '../views/HomeView.vue'
import DataBindingView from '../views/DataBindingView.vue'
const routes = [
{
path: '/',
name: 'home',
component: HomeView
},
{
path: '/about',
name: 'about',
// route level code-splitting
// this generates a separate chunk (about.[hash].js) for this route
// which is lazy-loaded when the route is visited.
component: () => import(/* webpackChunkName: "about" */ '../views/AboutView.vue')
},
{
path: '/databinding',
name: 'DataBindingView',
component: DataBindingView
}
]
const router = createRouter({
history: createWebHistory(process.env.BASE_URL),
routes
})
export default router
App.vue 파일에 링크 추가해야해
<router-link to="databinding">Data Binding</router-link>
<template>
<nav>
<router-link to="/">Home</router-link> |
<router-link to="/about">About</router-link>
<router-link to="databinding">Data Binding</router-link>
</nav>
<router-view/>
</template>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
}
nav {
padding: 30px;
}
nav a {
font-weight: bold;
color: #2c3e50;
}
nav a.router-link-exact-active {
color: #42b983;
}
</style>
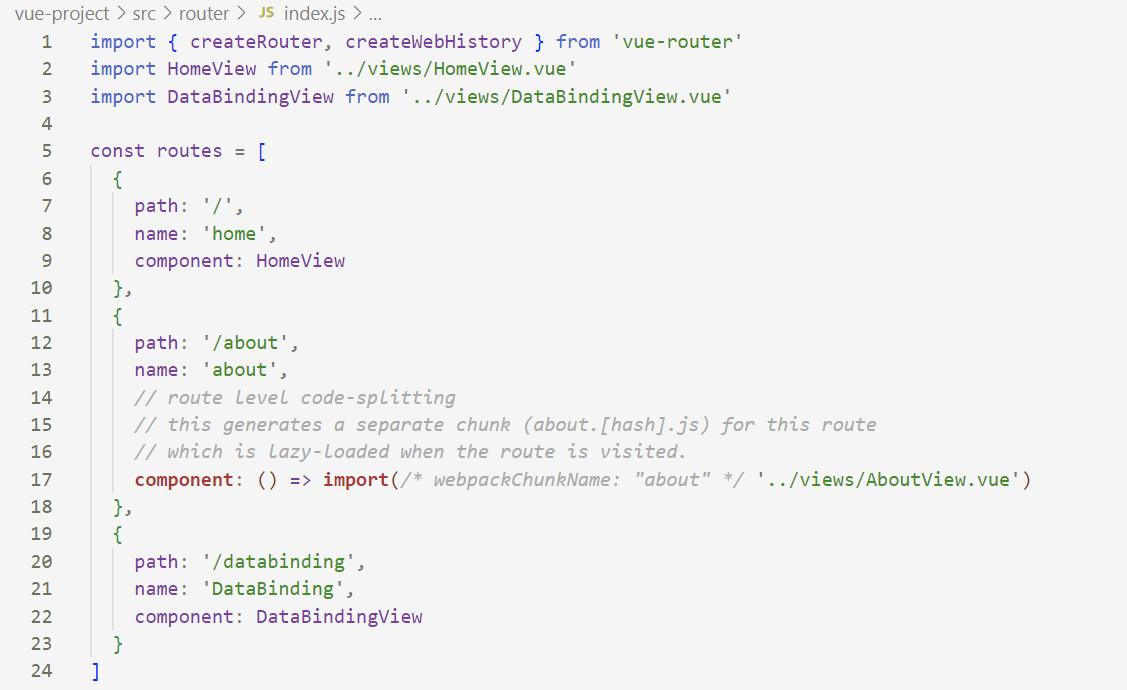
어떤게 뷰고 어떤게 컴포넌트인지 어떻게 구분할거야?
views는 view.vue라고 쓰자
import로 땡기는 건 컴포넌트! 뒤에 경로 있어야 해

path는 html 경로(주소에서만view빠졌어) => 링크는 패치 경로
component
html 바인딩
v-html
<template>
<div>
<div>{{htmlString}}</div>
<div v-html="htmlString"></div>
</div>
</template>
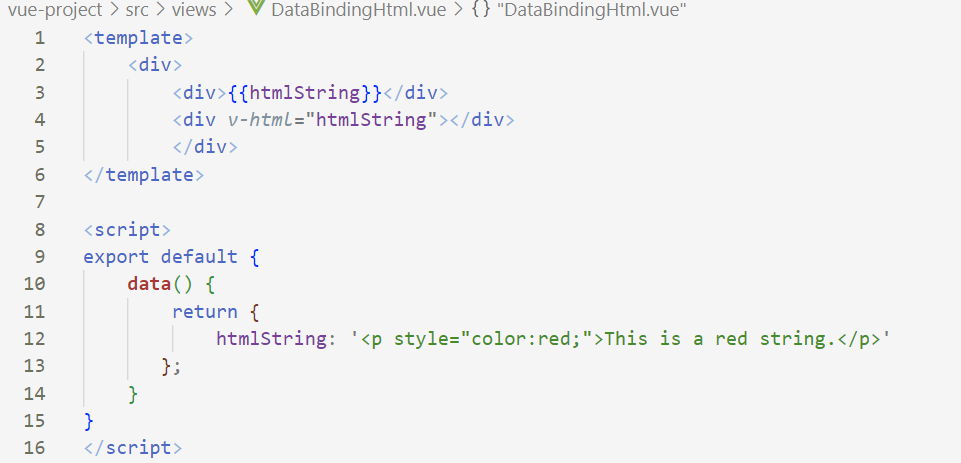
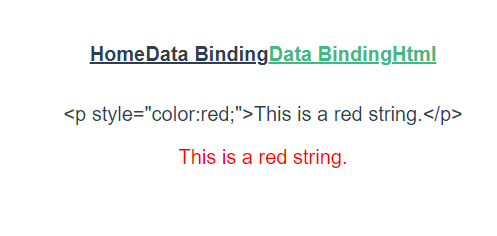
순서
일단 만들어
import 해 index.js 경로와 path, name, component
App.vue 링크 추가 html에 보여주기
Form 바인딩
v-model
* input type = text인 경우
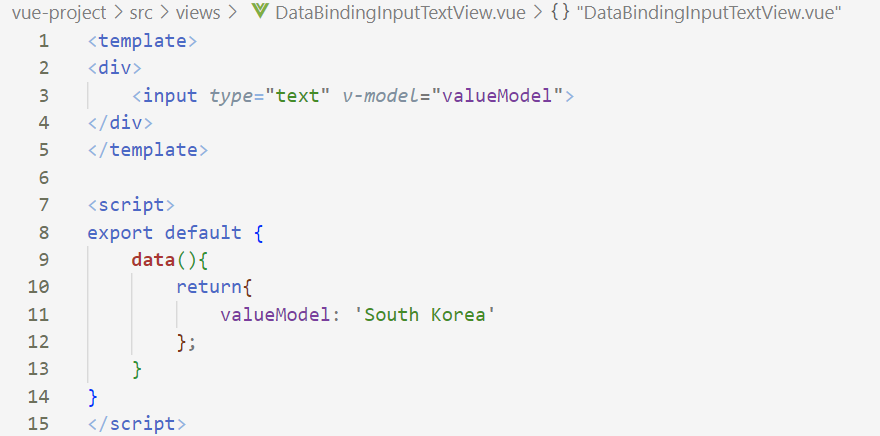
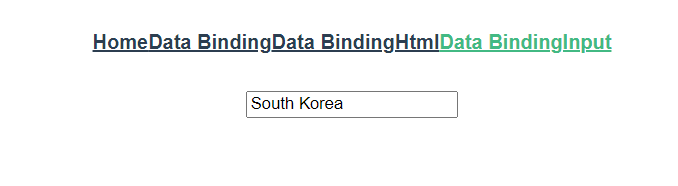
* input type =number인 경우
<template>
<div>
<input type="number" v-model.number="numberModel" />
</div>
</template>
<script>
export default {
data() {
return {
numberModel: 3
};
}
}
</script>
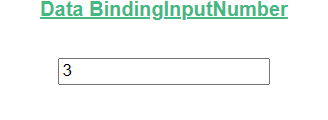
* textarea
<template>
<div>
<textarea v-model="message" />
</div>
</template>
<script>
export default {
data(){
return {
message: "여러 줄을 입력할 수 있는 textarea 입니다."
};
}
}
</script>
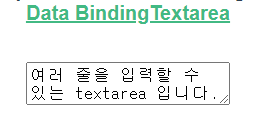
* checkbox
체크박스의 경우v model은 내부적으로 체크 박스의 checked속성을 사용
체크박스의 value 속성이 아닌 checked 속성
v-bind:value
<template>
<div>
<label><input type="checkbox" v-model="checked" true-value="yes" false-value="no"> {{checked}}</label>
</div>
</template>
<script>
export default {
data() {
return {
checked:true
};
}
}
</script>
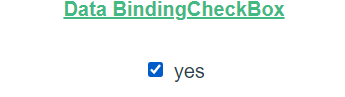
<template>
<div>
<label><input type="checkbox" value="서울" v-model="checked"> 서울</label>
<label><input type="checkbox" value="부산" v-model="checked"> 부산</label>
<label><input type="checkbox" value="제주" v-model="checked"> 제주</label>
<br>
<span>체크한 지역: {{ checked }}</span>
</div>
</template>
<script>
export default {
data(){
return{
checked: []
};
}
}
</script>
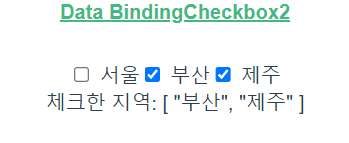
* Radio
하나밖에 선택 안돼서 배열로 만들지 않아
선택된 지역만 결과 나오게
<template>
<div>
<label><input type="radio" v-bind:value="radioValue1" v-model="picked"> 서울</label>
<label><input type="radio" v-bind:value="radioValue2" v-model="picked"> 부산</label>
<label><input type="radio" v-bind:value="radioValue3" v-model="picked"> 제주</label>
<br>
<span> 선택한 지역: {{ picked }}</span>
</div>
</template>
<script>
export default {
data() {
return{
picked: '',
radioValue1: '서울',
radioValue2: '부산',
radioValue3: '제주'
};
}
}
</script>
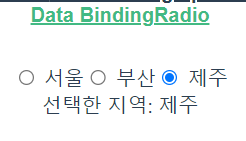
속성
v-bind:value
* img 객체의 src
<template>
<div>
<img v-bind:src="imgSrc" />
</div>
</template>
<script>
export default {
data() {
return {
imgSrc: "https://kr.vuejs.org/images/logo.png"
};
}
}
</script>
* button
<template>
<div>
<input type="text" v-model="textValue" />
<button type="button" v-bind:disabled="textValue ==''">Click</button>
</div>
</template>
<script>
export default {
data() {
return {
textValue: ""
};
}
}
</script>
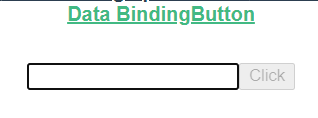
클래스 바인딩
Class
<template>
<div class="container" v-bind:class="{
'active': isActive, 'text-red': hasError }"
>Class Binding</div>
</template>
<script>
export default {
data() {
return {
isActive: true,
hasError: false
};
}
}
</script>
<style scoped>
.container {
width: 100%;
height: 200px;
}
.active{
background-color: yellow;
font-weight: bold;
}
.text-red {
color: red;
}
</style>
<template>
<div class="container" v-bind:class="{
'active': isActive, 'text-red': hasError }"
>Class Binding</div>
</template>
<script>
export default {
data() {
return {
isActive: true,
hasError: false
};
}
}
</script>
<style scoped>
.container {
width: 100%;
height: 200px;
}
.active{
background-color: yellow;
font-weight: bold;
}
.text-red {
color: red;
}
</style>
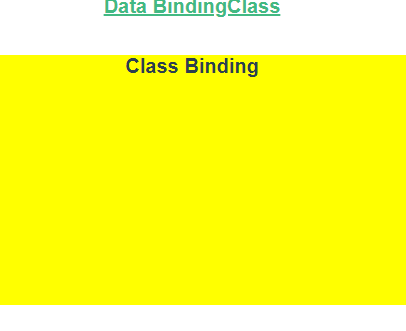
'MLOps 개발자 양성과정 > vue' 카테고리의 다른 글
[Day-42] vue 컴포넌트 심화 (0) | 2023.02.21 |
---|---|
[Day-41] vue 서버-데이터 바인딩 (0) | 2023.02.20 |
[Day-39] Vue 프레임워크 (0) | 2023.02.16 |